Java 단일 요소의 배열 Collections.singletonList vs Arrays.asList
반응형
나의 개발 친구 intelliJ가 친절하게 알려주었다. 단일 요소의 배열에는 asList()
대신 singletonList()
를 사용해달라고.
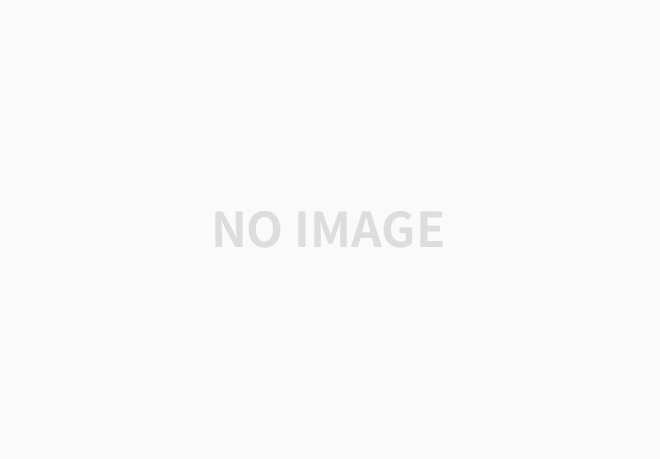
intelliJ가 괘 이러한 가이드를 주었는지 IntelliJ의 설명을 읽어 보자.
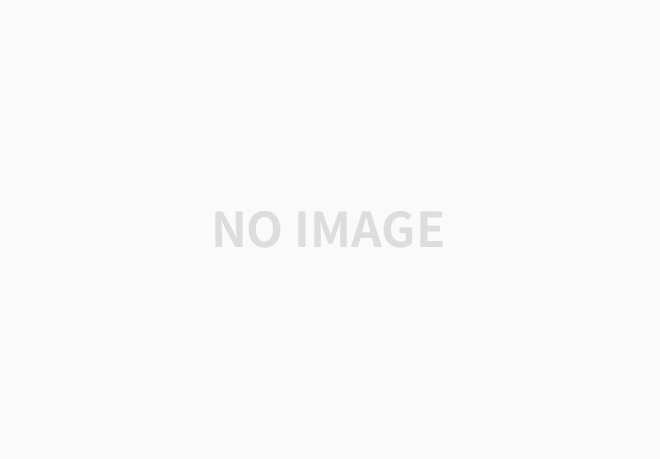
[요약]
메모리 절약을 위해서 요소가 없거나(empty) 하나인 경우에는 Collections.emptyList()
또는 Collections.singletonList()
를 사용해라.
Arrays.asList()는 배열의 요소가 한 개여도 Collections.singletonList()보다 사이즈가 크다는 것을 추측해볼 수 있다. 각 메소드에 대해 자세히 알아보자.
Collections.singletonList()
- 변경여부 : immutable (불변)
- 사이즈 : size가 1로 고정됨(지정된 단일 객체를 가르키는 주소값을 가지기 때문)
- 값 및 구조적 변경 시 UnsupportedOperationException 발생
Arrays.asList()
- 변경여부 : 값 변경 가능(set메소드가 허용)
- 사이즈 : 소유한 배열의 고정된 사이즈의 목록을 반환함
- 구조적 변경 시 UnsupportedOperationException 발생(ex 요소 추가, 삭제)
- ArrayList(AbstractList를 상속받은 중첩클래스)의 인스턴스를 반환함
- 해당 클래스는 set, indexOf, forEach, replaceAll 메소드를 구현하여 제공함
- 하지만 add 메소드 호출 시에는 AbstractList의 메소드를 호출하여 UnsupportedOperationException 반환
Immutable list
singletonList, asList와 직접적인 연관이 있는 내용은 아니지만, 알아 둘 필요가 있기에 함께 정리한다.
일반적으로 immutable list를 만들기 위해 Collections.unmodifiableList
를 사용한다. 이것은 특정한 list의 수정이 불가능한 view를 제공해주는 것이다. (엄밀히 말하면 immutable list가 반환되는 게 아님)
List<String> srcList = Arrays.asList("사과", "망고", "바나나"); var fruits = new ArrayList<>(srcList); var unmodifiableList = Collections.unmodifiableList(fruits); fruits.set(0, "수박"); var modFruit = unmodifiableList.get(0); System.out.println(modFruit); // prints 수박
unmodifiableList는 원래의 객체를 가르키고 있는 수정이 불가한 collections일 뿐, 원래의 객체가 수정되면 unmodifiableList가 보여주는 값도 따라서 바뀐다.
정말 immutable한 list를 만들고 싶은 경우, 두 가지 방법이 있다. (Java 10 이후)
var unmodifiableList = List.copyOf(srcList);
var unmodifiableList = srcList.stream().collect(Collectors.toUnmodifiableList());
이 경우, 값이 '수박'으로 바뀌지 않고 '사과'로 유지된다.
As per doc of Java 10:
The List.of and List.copyOf static factory methods provide a convenient way to create unmodifiable lists. The List instances created by these methods have the following characteristics:They are unmodifiable. Elements cannot be added, removed, or replaced. Calling any mutator method on the List will always cause UnsupportedOperationException to be thrown. However, if the contained elements are themselves mutable, this may cause the List's contents to appear to change.They disallow null elements. Attempts to create them with null elements result in NullPointerException.They are serializable if all elements are serializable.The order of elements in the list is the same as the order of the provided arguments, or of the elements in the provided array.They are value-based. Callers should make no assumptions about the identity of the returned instances. Factories are free to create new instances or reuse existing ones. Therefore, identity-sensitive operations on these instances (reference equality (==), identity hash code, and synchronization) are unreliable and should be avoided.They are serialized as specified on the Serialized Form page.
이번 내용은 아래 게시글을 정리한 내용이다.
반응형
댓글을 사용할 수 없습니다.